Another simple responsive Bootstrap 5 testimonial slider using the Bootstrap carousel with multiple items.
Final output:
“Some quick example text to build on the card title and make up the bulk of the card’s content.”
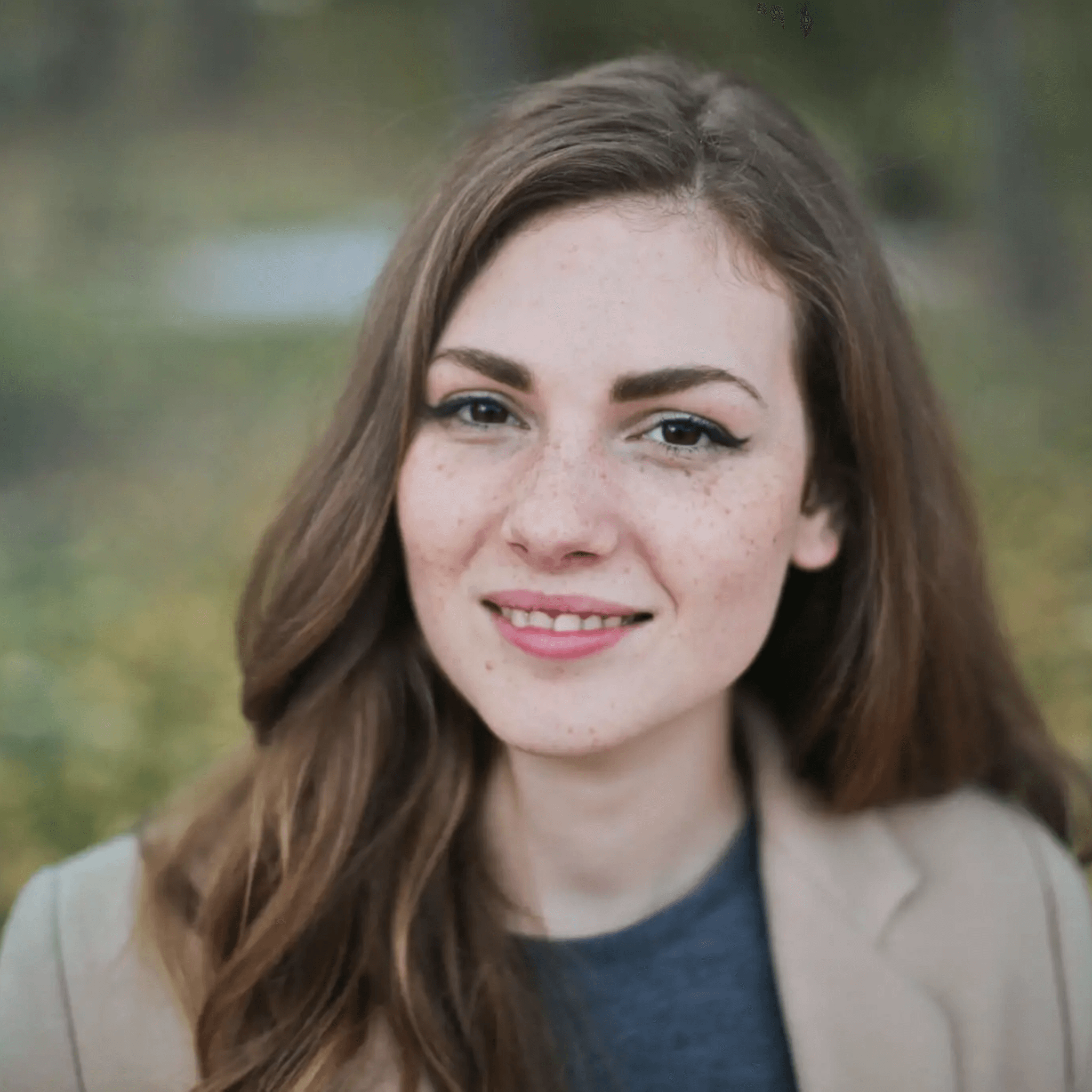
Jane Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
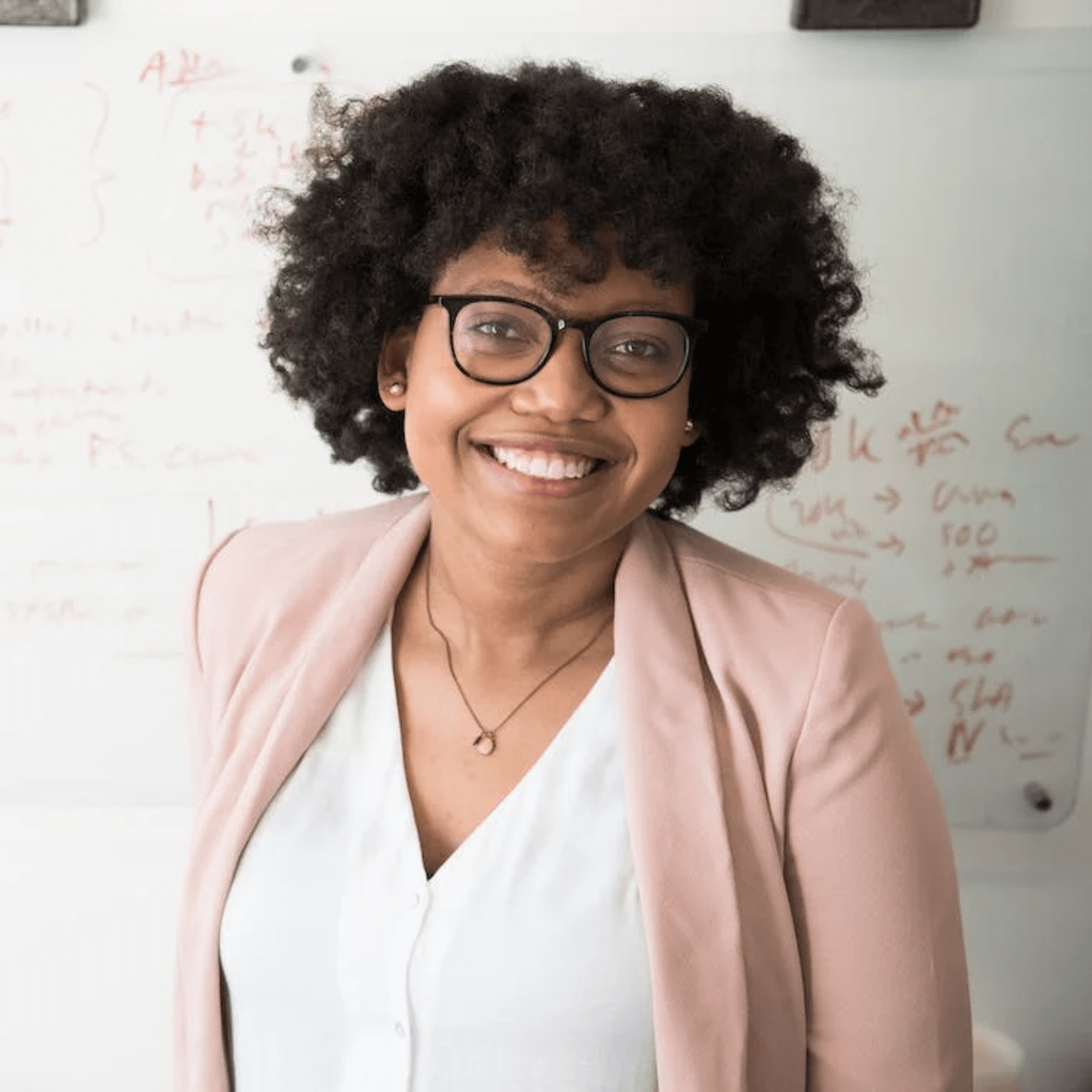
June Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
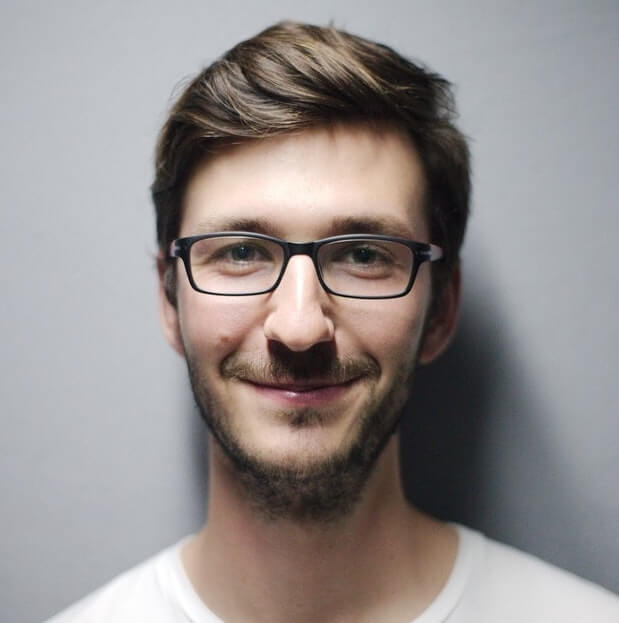
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
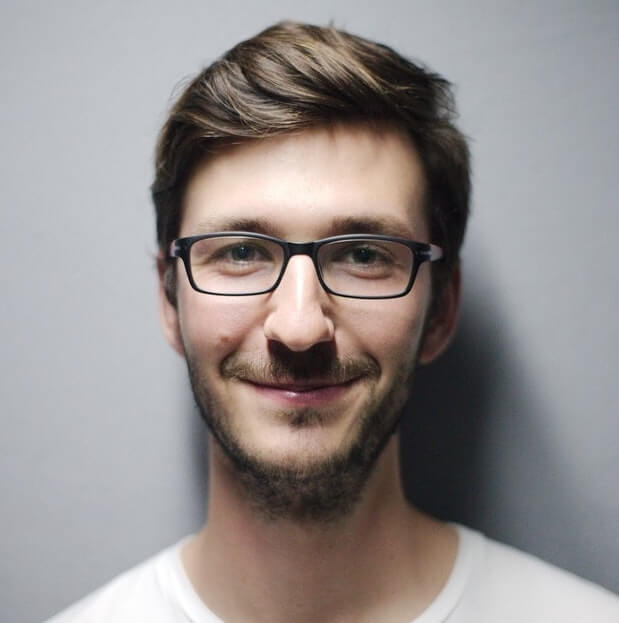
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
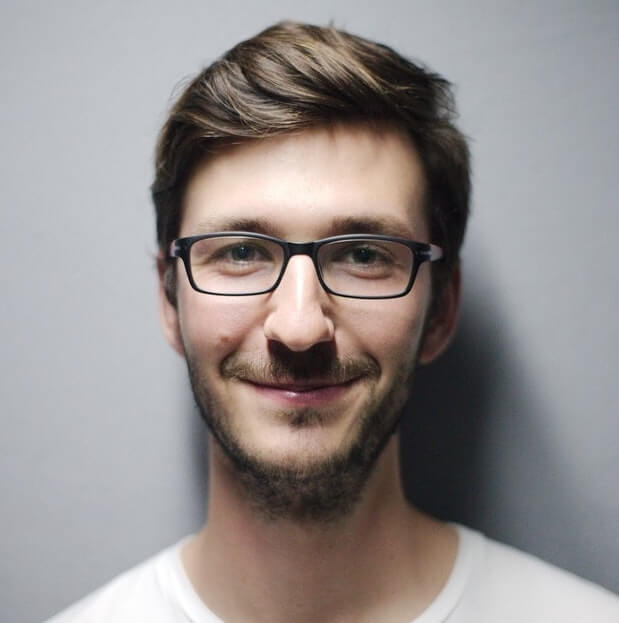
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
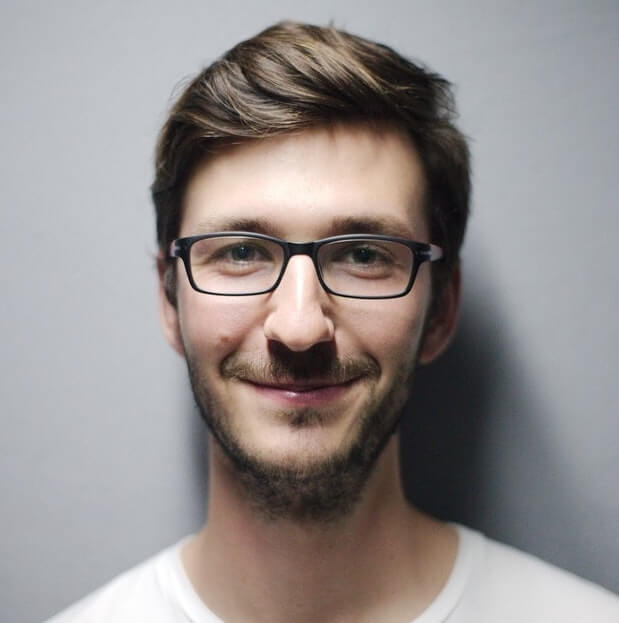
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
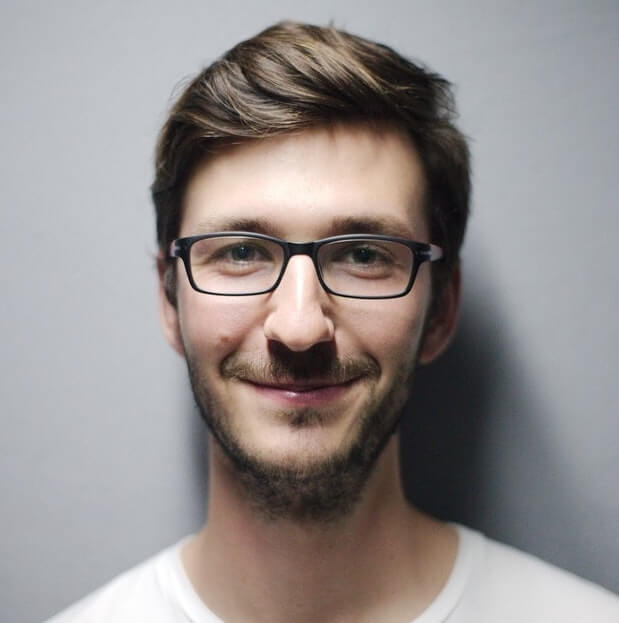
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
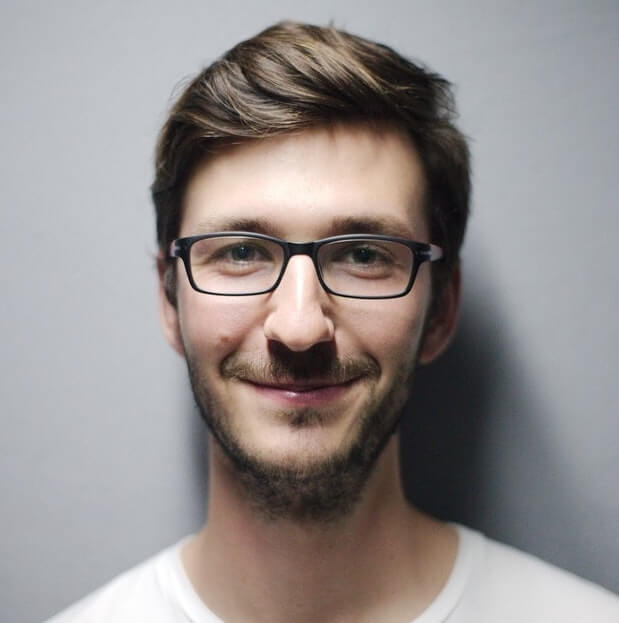
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
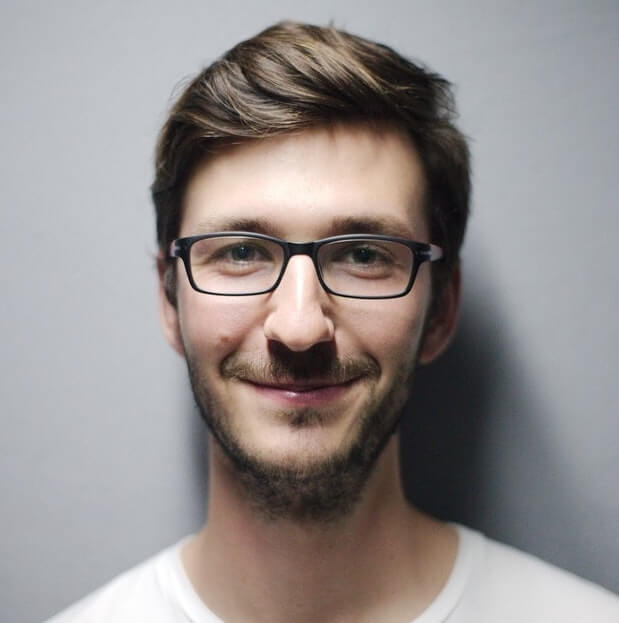
John Doe
CEO, Example Company1. I’ll be using the Bootstrap 5 Carousel Multiple Items slider from a previous post for the Bootstrap testimonial slider.
(Rerun when changing sizes)
See the Pen Bootstrap carousel multiple items increment by 1 (jQuery) by Coding Yaar (@codingyaar) on CodePen.
For Bootstrap 5.2+, add wrap: false to stop the continuous cycle.
var carousel = new bootstrap.Carousel(multipleCardCarousel, {
interval: false,
wrap: false,
});
2. Install Bootstrap icons. Add the quotes icon inside the card. Wrap it in a div and use the display class to increase its size. Change the font color using the class text-body-tertiary.
<div class="display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
Remove the button. Place the image and the card title inside another div in the card body below the card-text. Let’s remove the img-wrapper div and use even-size images. Use flex to align items. And add some padding on top.
<div class="d-flex align-items-center pt-2">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Jane Doe</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
Remove the img-wrapper and img styles and use this instead. Specify the image width and height. For a circular image, set the border radius to 50% and overflow to hidden. Add some right margin.
.carousel img {
width: 70px;
max-height: 70px;
border-radius: 50%;
margin-right: 1rem;
overflow: hidden;
}
This is how the testimonial card HTML should look:
<div class="card">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">Some quick example text to build on the card title and make up the bulk of
the card's content.</p>
<div class="d-flex align-items-center pt-2">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Jane Doe</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
Output:
Some quick example text to build on the card title and make up the bulk of the card’s content.
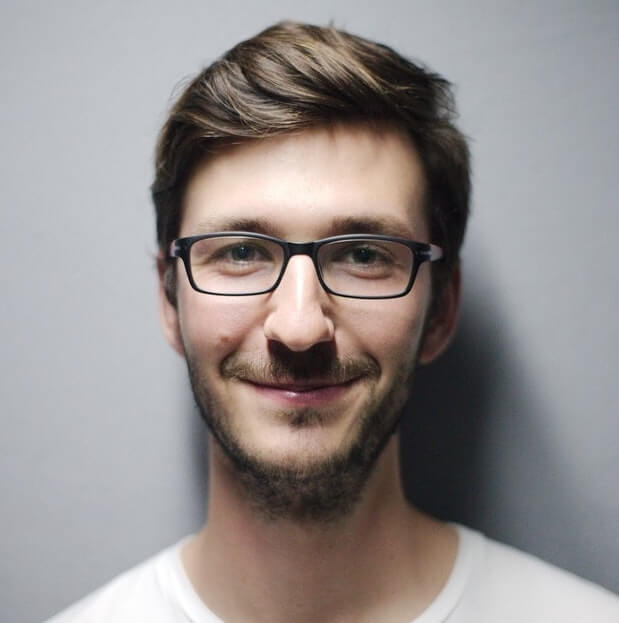
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
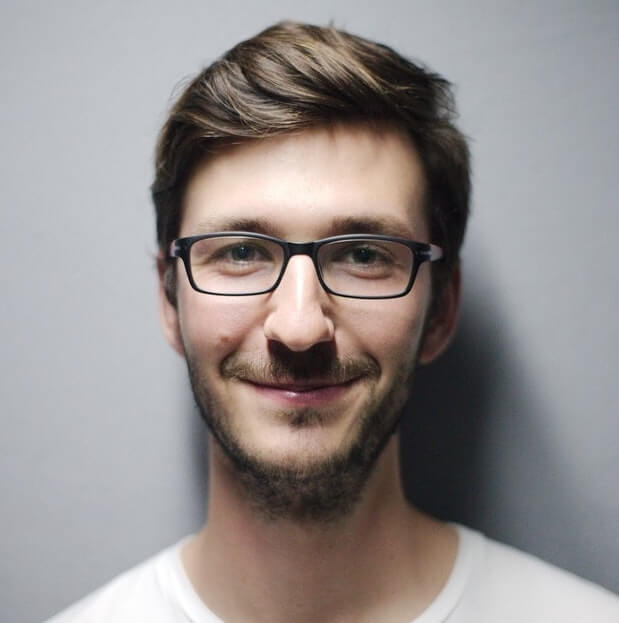
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
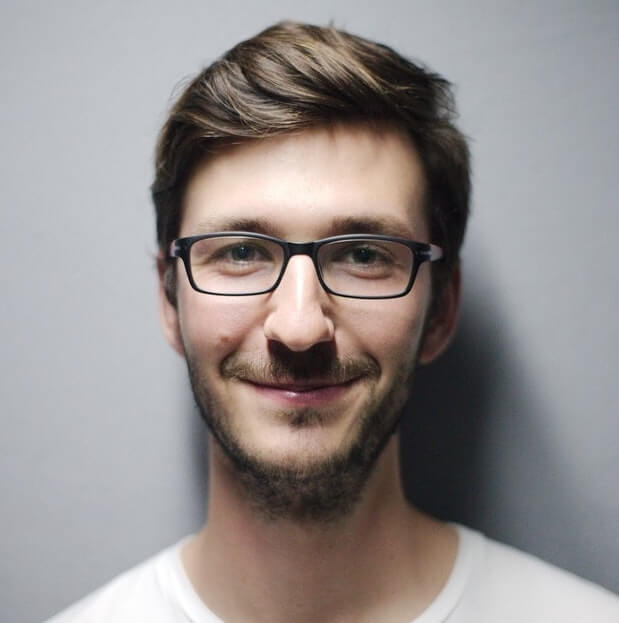
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
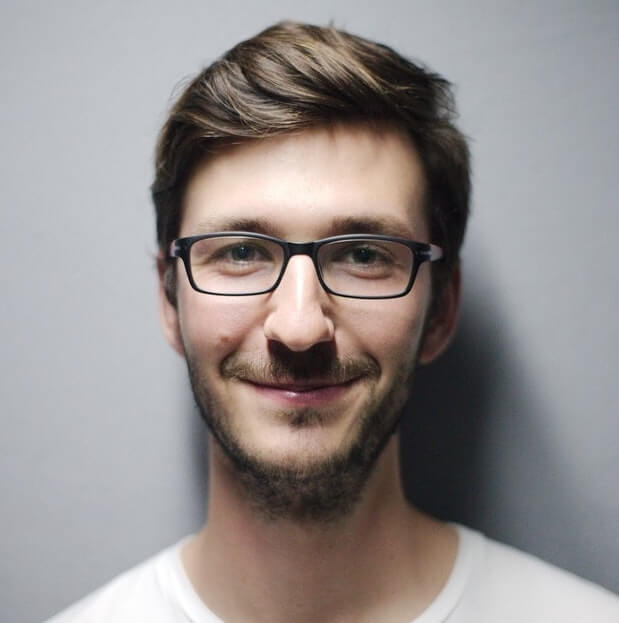
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
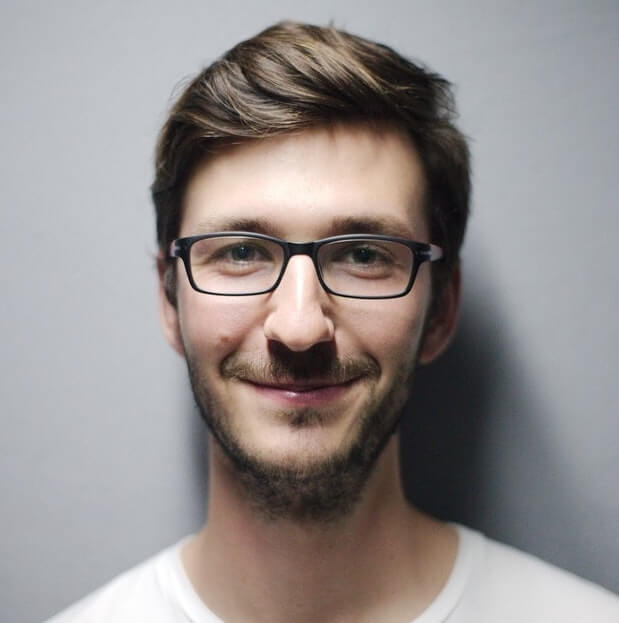
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
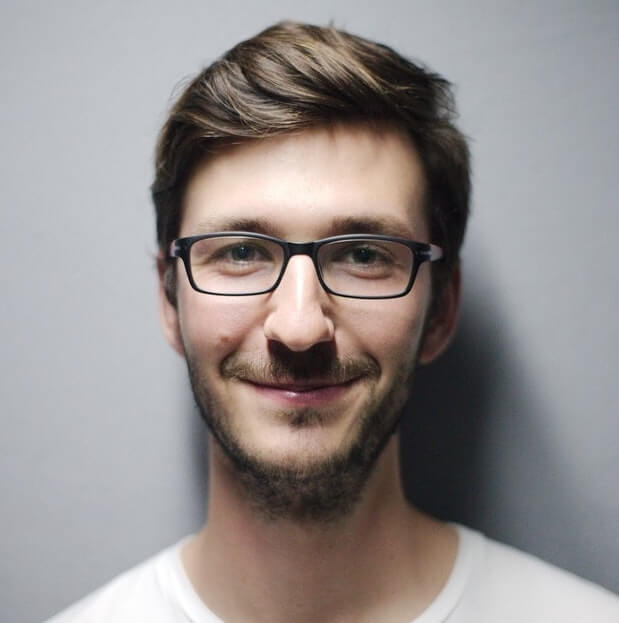
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
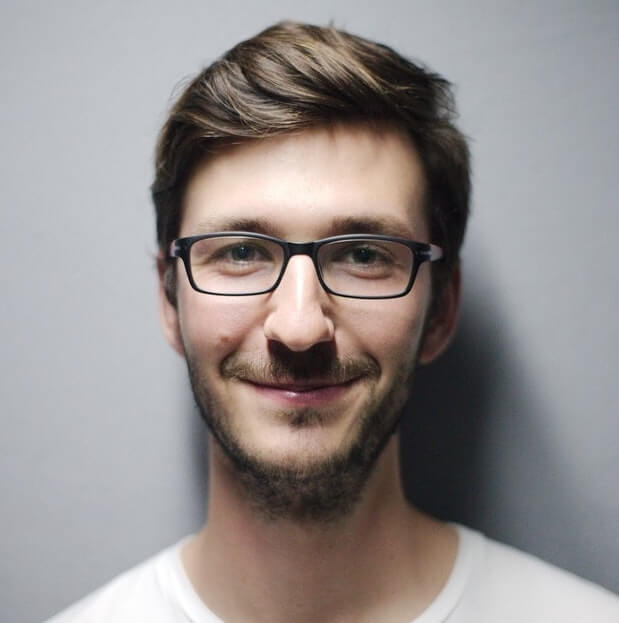
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
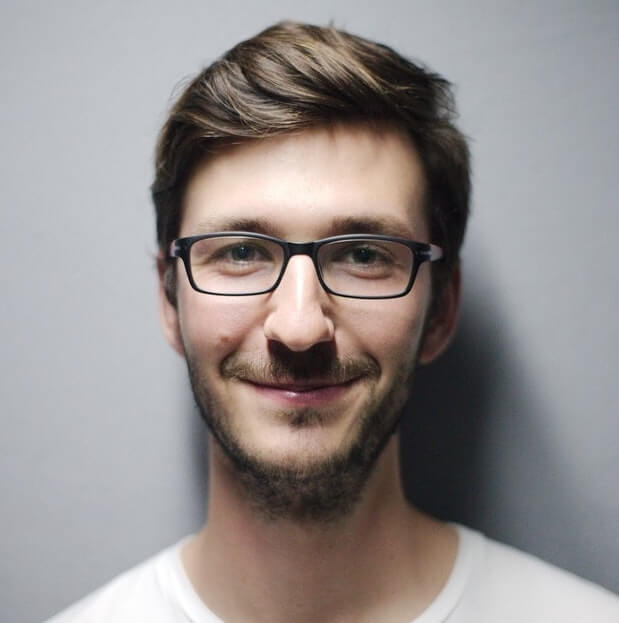
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
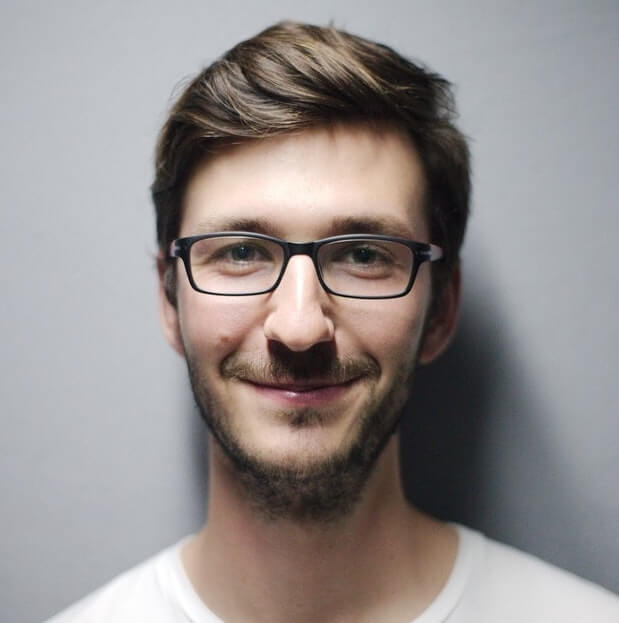
John Doe
CEO, Example Company3. Let’s display three cards only on large screens. For screens with a width of 576px to 1024px, display only two cards.
@media screen and (min-width: 576px) {
.carousel-item {
display: block;
margin-right: 0;
flex: 0 0 calc(100% / 2);
}
}
@media screen and (min-width: 1024px) {
.carousel-item {
flex: 0 0 calc(100% / 3);
}
}
Let’s place the carousel controls away from the cards(currently overlapping).
.carousel-inner {
display: flex;
width: 90%;
margin-inline: auto;
padding: 1em 0;
overflow: hidden;
}
Output:
Some quick example text to build on the card title and make up the bulk of the card’s content.
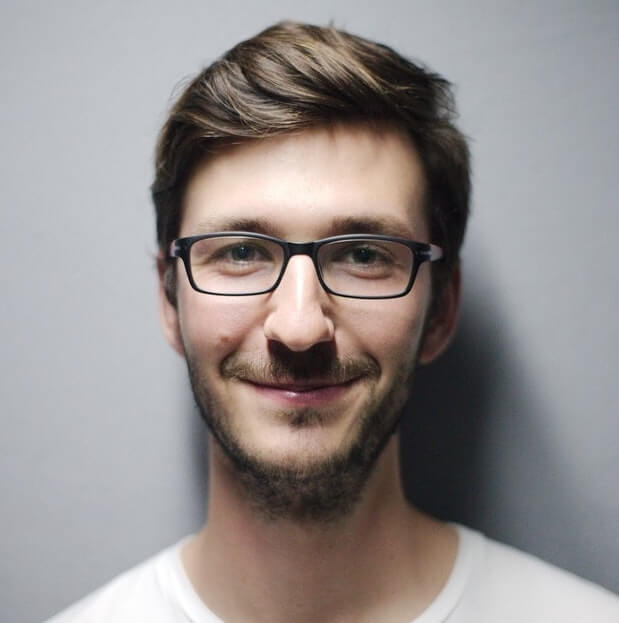
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
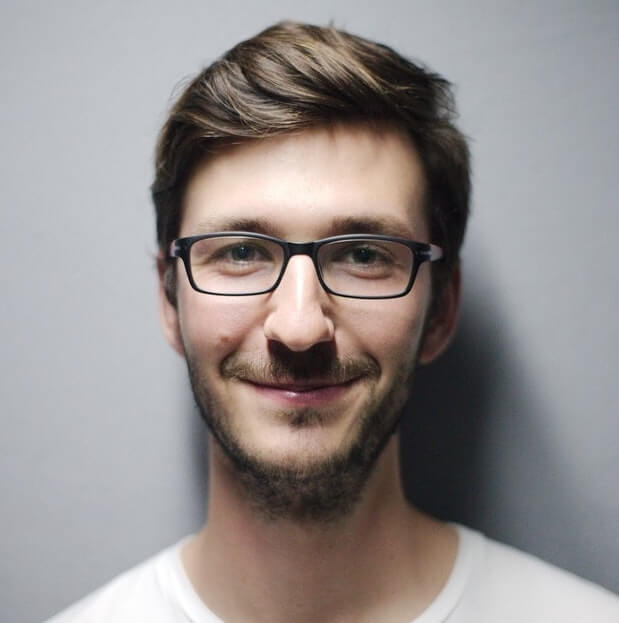
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
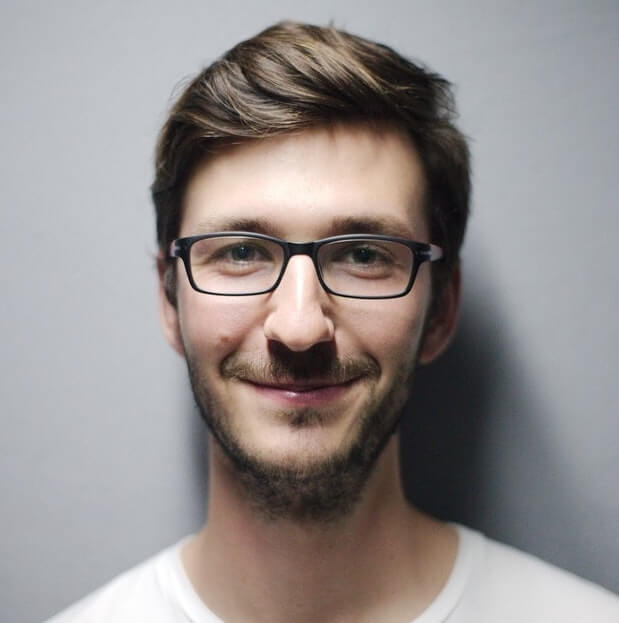
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
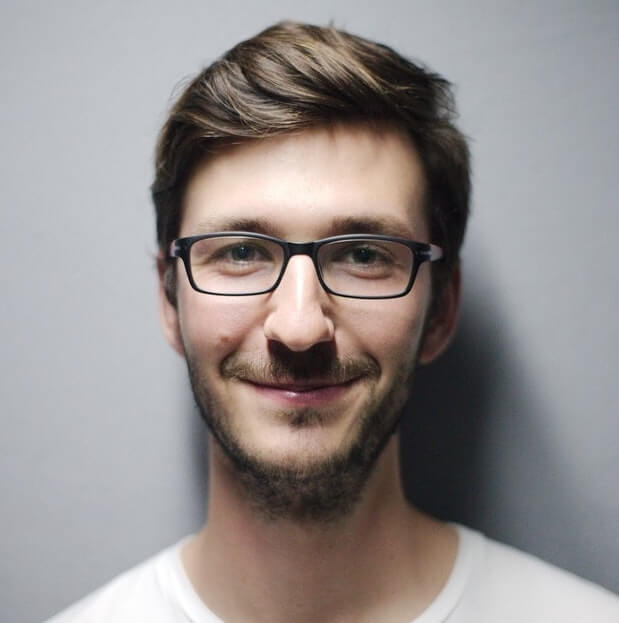
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
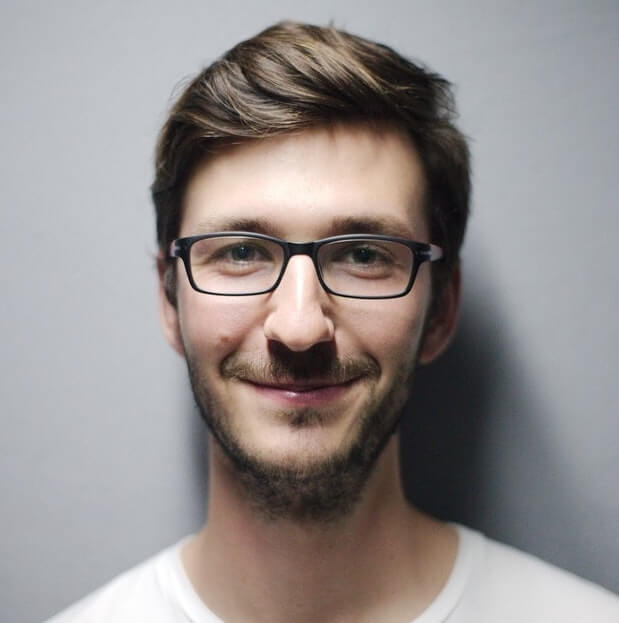
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
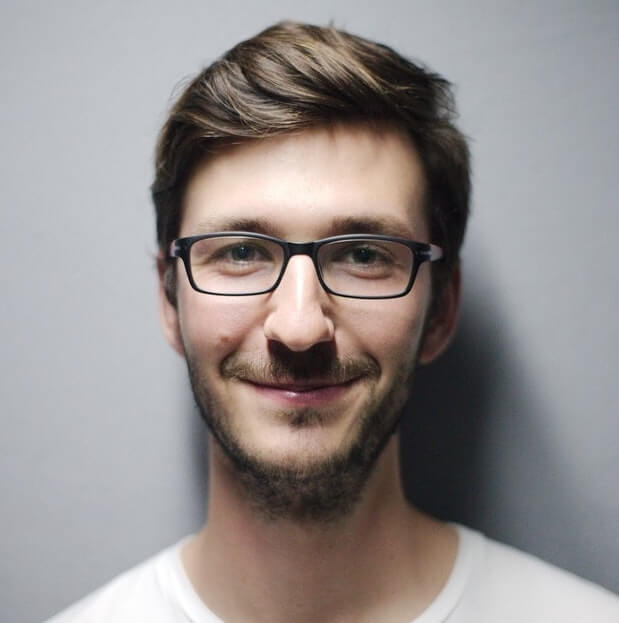
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
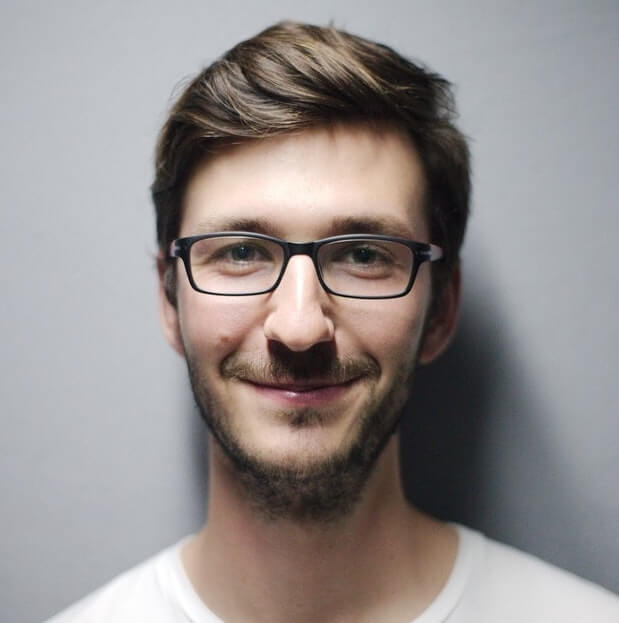
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
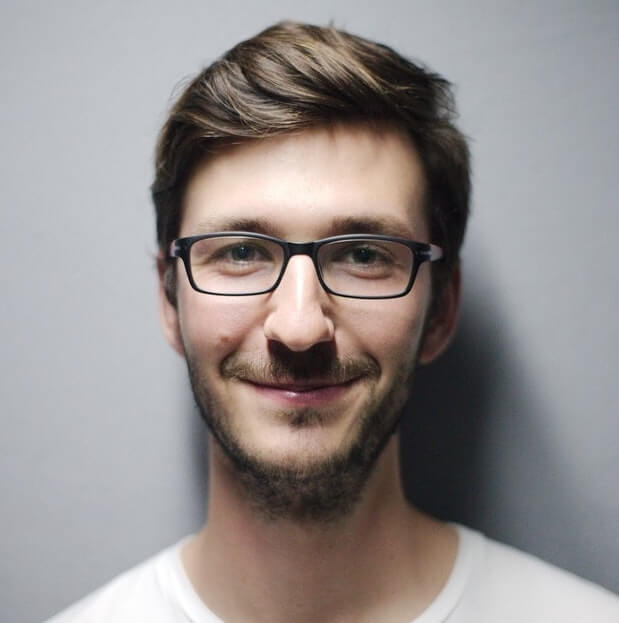
John Doe
CEO, Example CompanySome quick example text to build on the card title and make up the bulk of the card’s content.
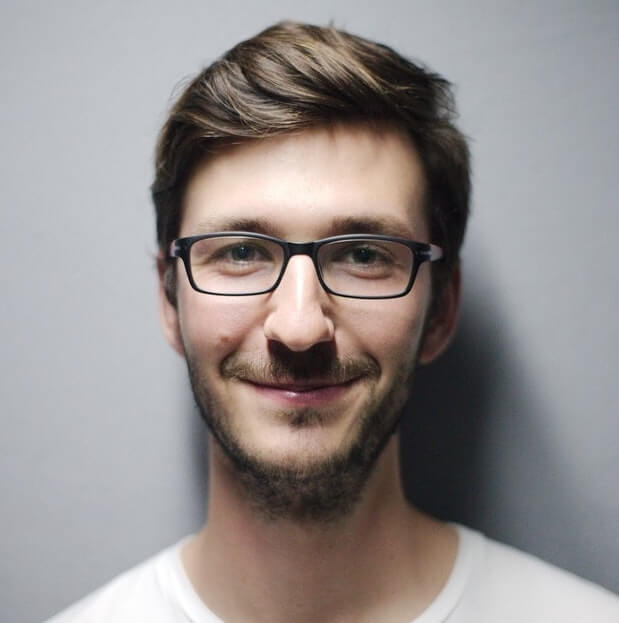
John Doe
CEO, Example Company4. You can change the box shadow for the card. I’m changing to a smaller shadow using the Bootstrap class shadow-sm. Set the border to 0. Change the border-radius too(rounded-3).
<div class="card shadow-sm border-0 rounded-3">
Change the background color for the controls.
.carousel-control-prev,
.carousel-control-next {
width: 3rem;
height: 3rem;
background-color: grey;
border-radius: 50%;
top: 50%;
transform: translateY(-50%);
}
Output:
“Some quick example text to build on the card title and make up the bulk of the card’s content.”
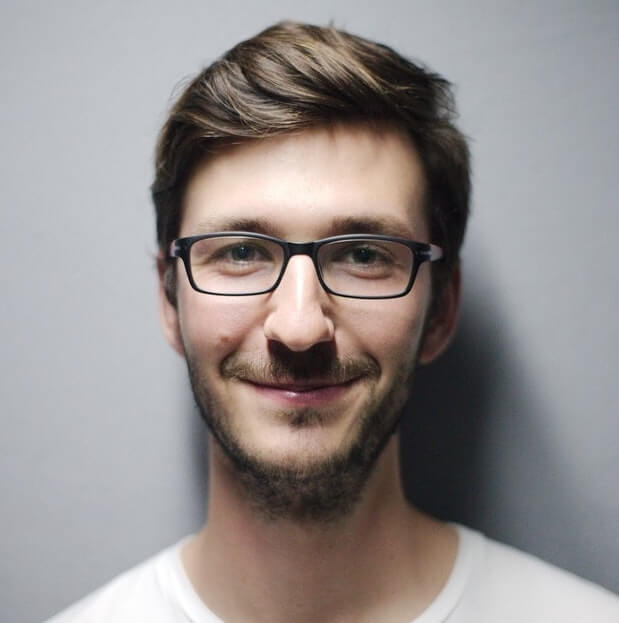
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
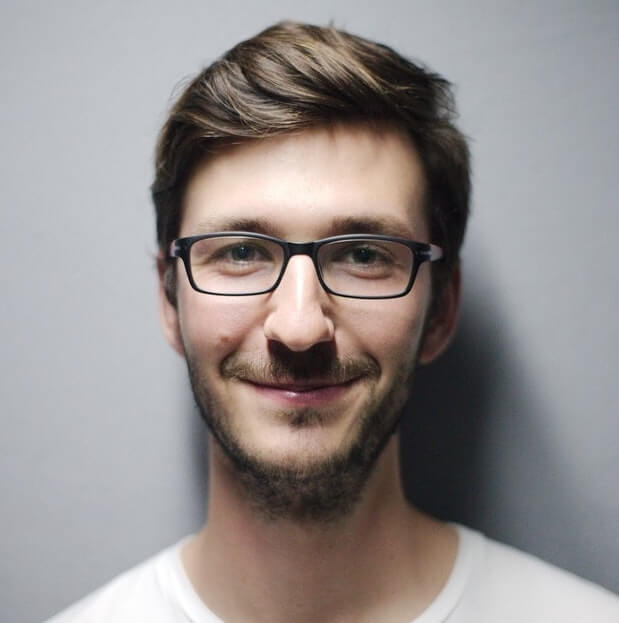
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
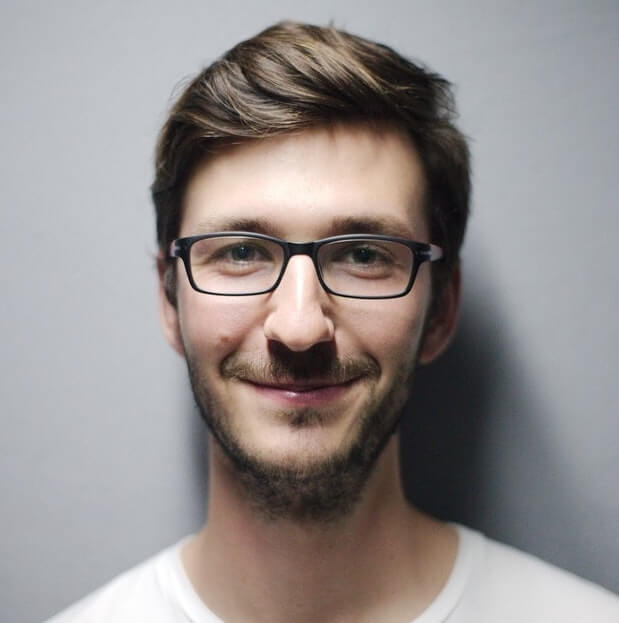
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
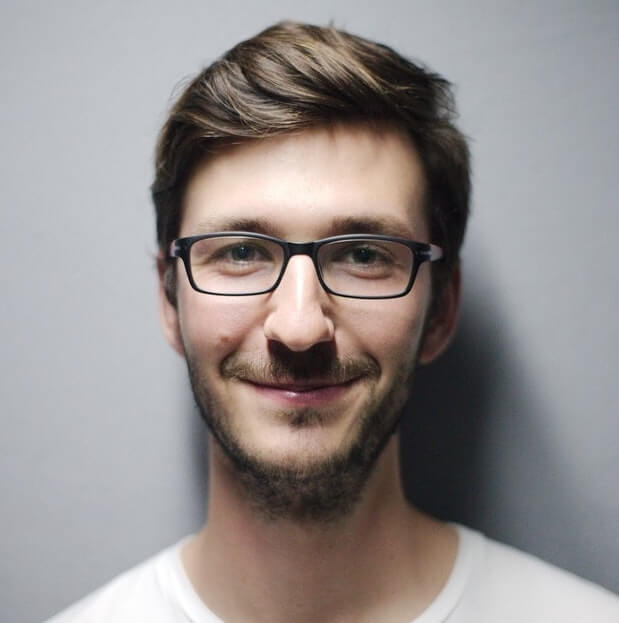
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
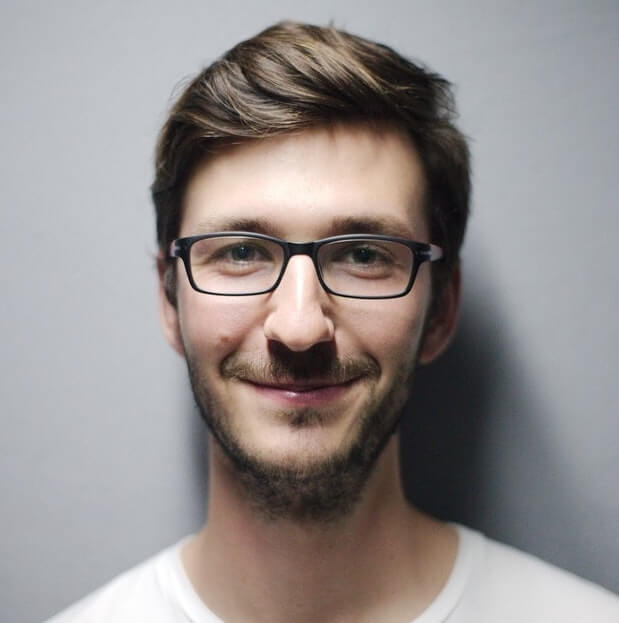
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
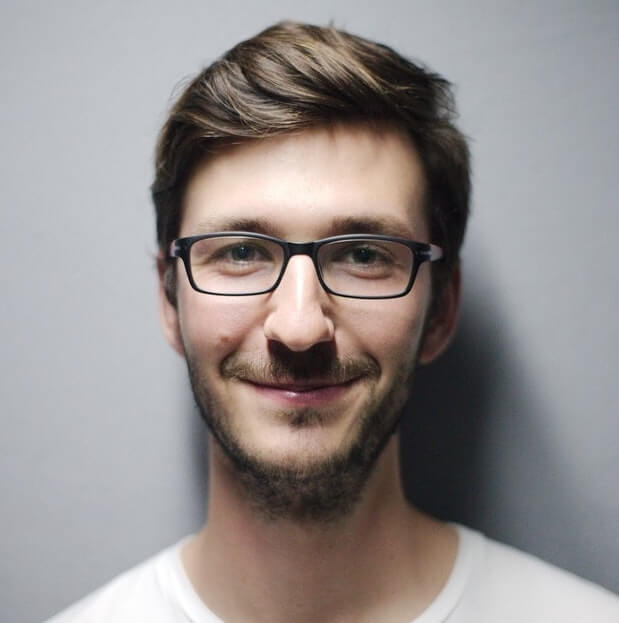
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
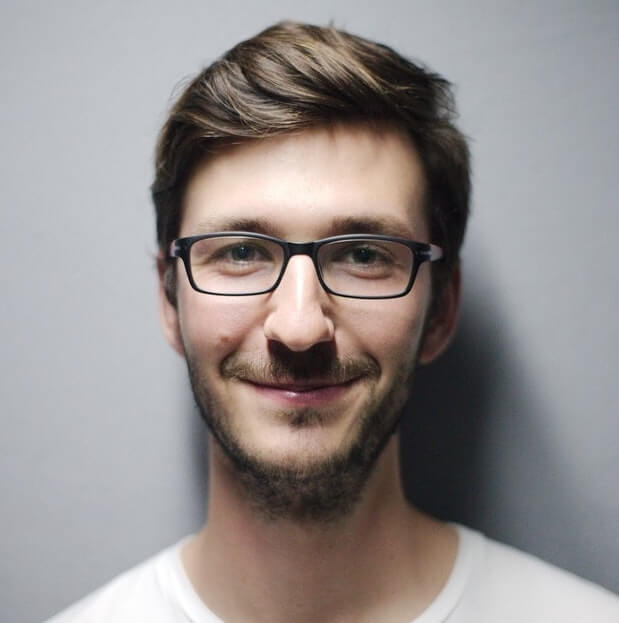
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
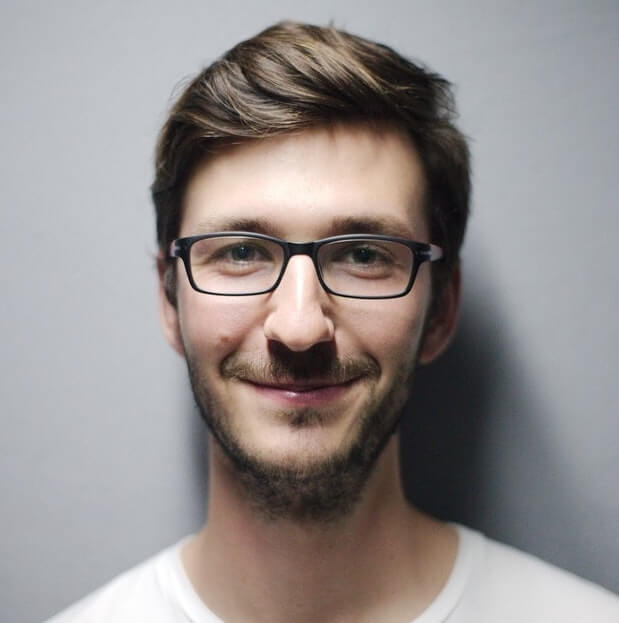
John Doe
CEO, Example Company“Some quick example text to build on the card title and make up the bulk of the card’s content.”
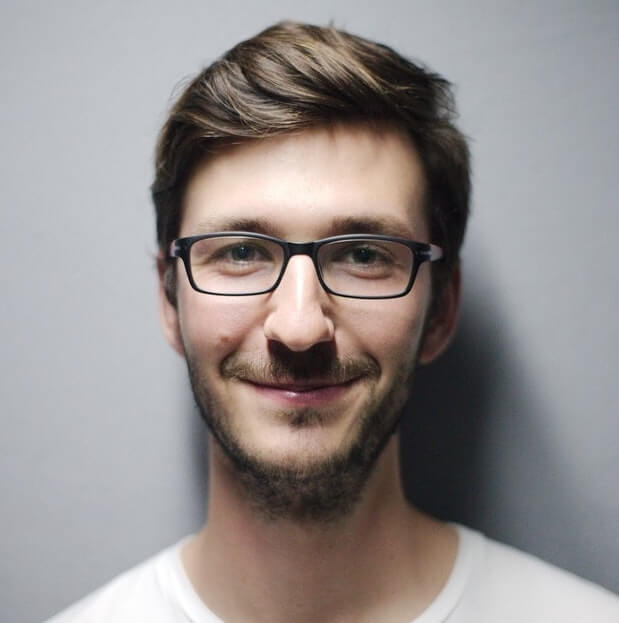
John Doe
CEO, Example CompanyVideo tutorial for Bootstrap 5 Testimonial Slider #2:
Final Output Code for Bootstrap 5 Testimonial Carousel Slider #2:
HTML
<div class="container bg-body-tertiary py-3">
<div id="testimonialCarousel" class="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Jane Doe</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">June Doe</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">John Doe</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 4</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 5</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 6</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 7</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 8</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
<div class="carousel-item">
<div class="card shadow-sm rounded-3 p-2">
<div class="quotes display-2 text-body-tertiary">
<i class="bi bi-quote"></i>
</div>
<div class="card-body">
<p class="card-text">"Some quick example text to build on the card title and make up the
bulk of
the card's content."</p>
<div class="d-flex align-items-center pt-4">
<img src="..." alt="">
<div>
<h5 class="card-title fw-bold">Card title 9</h5>
<span class="text-secondary">CEO, Example Company</span>
</div>
</div>
</div>
</div>
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#testimonialCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#testimonialCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
</div>
CSS
.carousel img {
width: 70px;
max-height: 70px;
border-radius: 50%;
margin-right: 1rem;
overflow: hidden;
}
.carousel-inner {
padding: 1em;
}
@media screen and (min-width: 576px) {
.carousel-inner {
display: flex;
width: 90%;
margin-inline: auto;
padding: 1em 0;
overflow: hidden;
}
.carousel-item {
display: block;
margin-right: 0;
flex: 0 0 calc(100% / 2);
}
}
@media screen and (min-width: 1024px) {
.carousel-item {
display: block;
margin-right: 0;
flex: 0 0 calc(100% / 3);
}
}
.carousel .card {
margin: 0 0.5em;
border: 0;
}
.carousel-control-prev,
.carousel-control-next {
width: 3rem;
height: 3rem;
background-color: grey;
border-radius: 50%;
top: 50%;
transform: translateY(-50%);
}
jQuery
const multipleItemCarousel = document.querySelector("#testimonialCarousel");
if (window.matchMedia("(min-width:576px)").matches) {
const carousel = new bootstrap.Carousel(multipleItemCarousel, {
interval: false,
});
var carouselWidth = $(".carousel-inner")[0].scrollWidth;
var cardWidth = $(".carousel-item").width();
var scrollPosition = 0;
$(".carousel-control-next").on("click", function () {
if (scrollPosition < carouselWidth - cardWidth * 3) {
console.log("next");
scrollPosition = scrollPosition + cardWidth;
$(".carousel-inner").animate({ scrollLeft: scrollPosition }, 800);
}
});
$(".carousel-control-prev").on("click", function () {
if (scrollPosition > 0) {
scrollPosition = scrollPosition - cardWidth;
$(".carousel-inner").animate({ scrollLeft: scrollPosition }, 800);
}
});
} else {
$(multipleItemCarousel).addClass("slide");
}
If you have any doubts or stuck somewhere, you can reach out through Coding Yaar's Discord server.