How to create a simple responsive HTML CSS product card.
Final output:
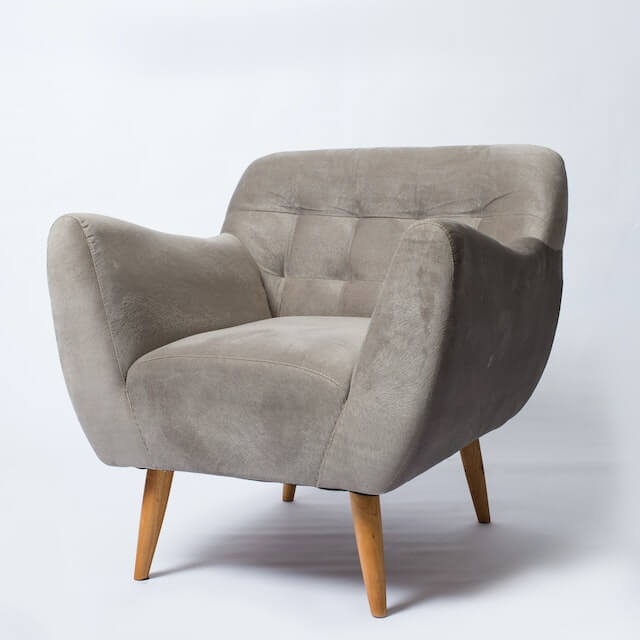
Product Name
1. Let’s start by adding a div with a classname product-card. Add the product image and the product name inside it.
<div class="product-card">
<img src="..." alt="">
<h4>Product Name</h4>
</div>
Using another div inside the product-card, add a price and a little add-to-cart button.
<div class="product-card">
<img src="..." alt="">
<h4>Product Name</h4>
<div>
<span>$299</span>
<button>+</button>
</div>
</div>
Output:
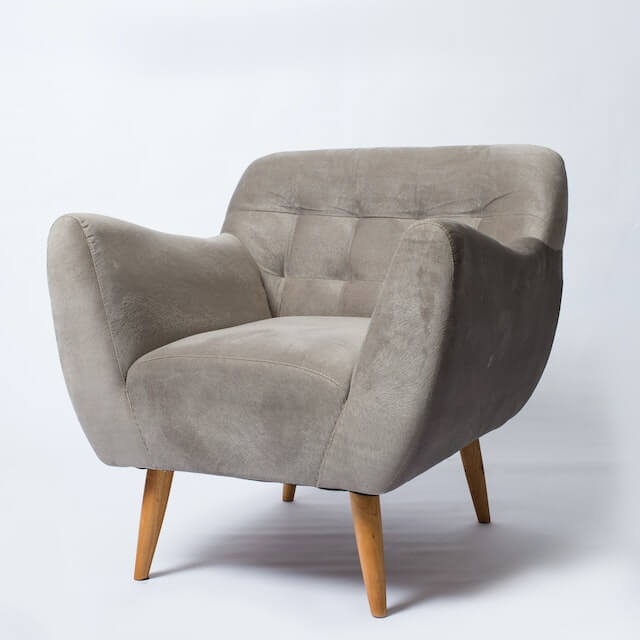
Product Name
2. For the product card, set the max-width to 12em, and for the image set it to 100%.
.product-card {
max-width: 12em;
}
.product-card img {
max-width: 100%;
}
Output:
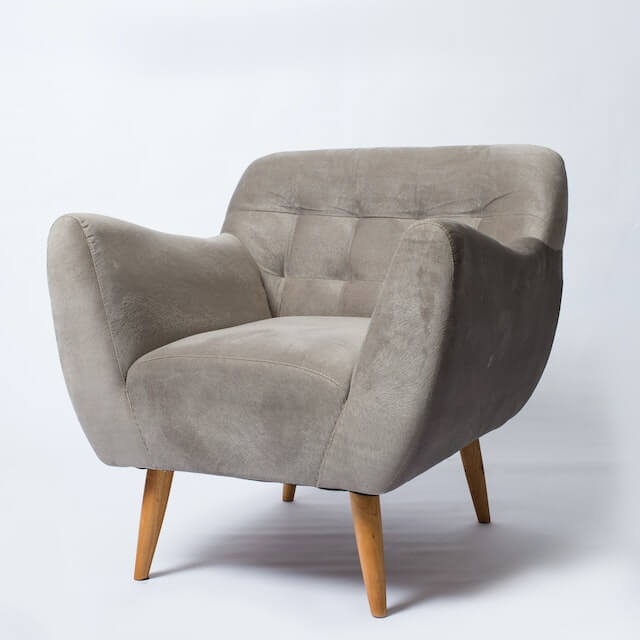
Product Name
3. Set a background color for the card, along with some padding and a box-shadow.
Also, change the font family for the card.
.product-card {
background-color: #fff;
padding: 1em;
box-shadow: 0 5px 5px #e1e1e1;
font-family: Arial, Helvetica, sans-serif;
}
For the product name, set the top and bottom margins to 0.5em and increase the font size to 1.3em. Set the font weight to bold.
.product-card h4 {
font-size: 1.3em;
margin: 0.5em 0;
font-weight: bold;
}
Output:
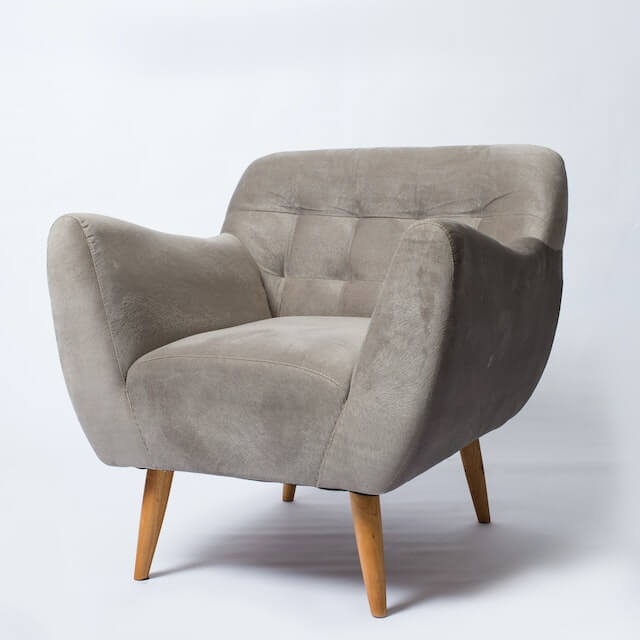
Product Name
4. Now for the div at the bottom, increase its font size and use CSS flexbox to align its content.
.product-card div {
font-size: 1.2em;
display: flex;
justify-content: space-between;
align-items: center;
}
Let’s style the button. Change the background and the font color. Set some fixed width and height and align the icon to the center using CSS flexbox properties.
.product-card button {
background-color: #000;
color: #fff;
border: none;
border-radius: 50%;
width: 25px;
height: 25px;
font-size: 1em;
display: flex;
justify-content: center;
align-items: center;
}
Output:
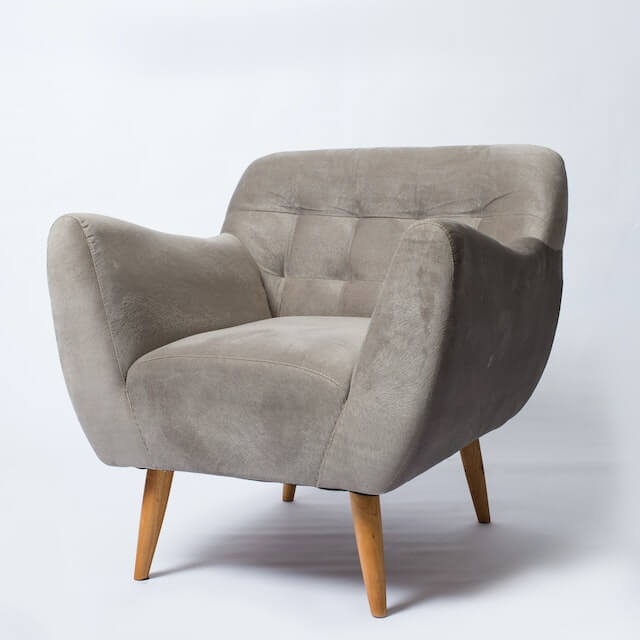
Product Name
5. On card hover, change the background and the font color.
.product-card:hover {
background-color: lightslategray;
color: #fff;
}
Let’s switch the colors for the button too.
.product-card:hover button {
color: #000;
background-color: #fff;
}
Output:
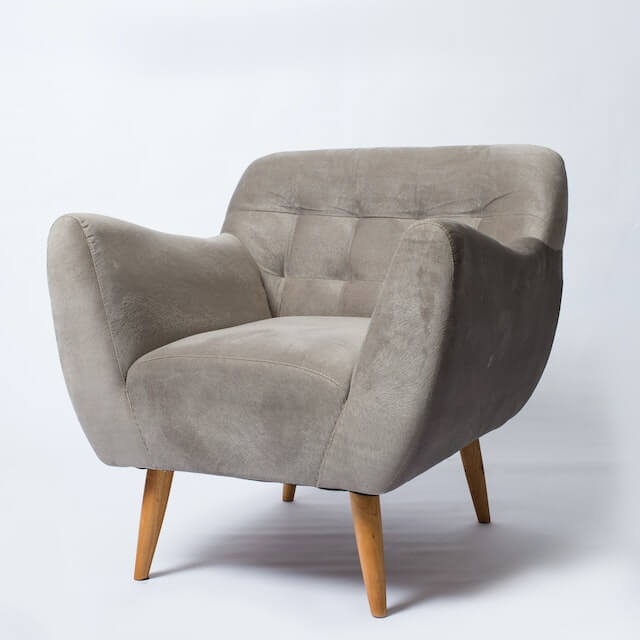
Product Name
6. The font size will be too large for the smaller screens. Add a media query for the smaller screens and reduce the font size for the product card.
@media screen and (max-width: 576px) {
.product-card {
font-size: 0.9em;
}
}
Output:
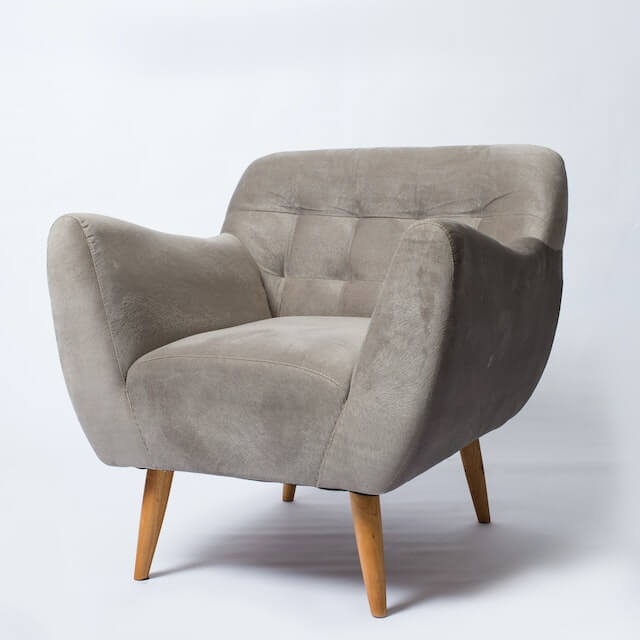
Product Name
Final Output Code for Responsive HTML CSS Product Card:
HTML
<div class="product-card">
<img src="..." alt="">
<h4>Product Name</h4>
<div>
<span>$299</span>
<button>+</button>
</div>
</div>
CSS
.product-card {
max-width: 12em;
background-color: #fff;
padding: 1em;
box-shadow: 0 5px 5px #e1e1e1;
font-family: Arial, Helvetica, sans-serif;
}
.product-card img {
max-width: 100%;
}
.product-card h4 {
font-size: 1.3em;
margin: 0.5em 0;
font-weight: bold;
}
.product-card div {
font-size: 1.2em;
display: flex;
justify-content: space-between;
align-items: center;
}
.product-card button {
background-color: #000;
color: #fff;
border: none;
border-radius: 50%;
width: 25px;
height: 25px;
font-size: 1em;
display: flex;
justify-content: center;
align-items: center;
}
.product-card:hover {
background-color: lightslategray;
color: #fff;
}
.product-card:hover button {
color: #000;
background-color: #fff;
}
@media screen and (max-width: 576px) {
.product-card {
font-size: 0.9em;
}
}
Video tutorial for Responsive HTML CSS Product Card:
If you have any doubts or stuck somewhere, you can reach out through Coding Yaar's Discord server.