Here’s a simple way to create a responsive Bootstrap 5 Modal Form with a dark background.
Final output:
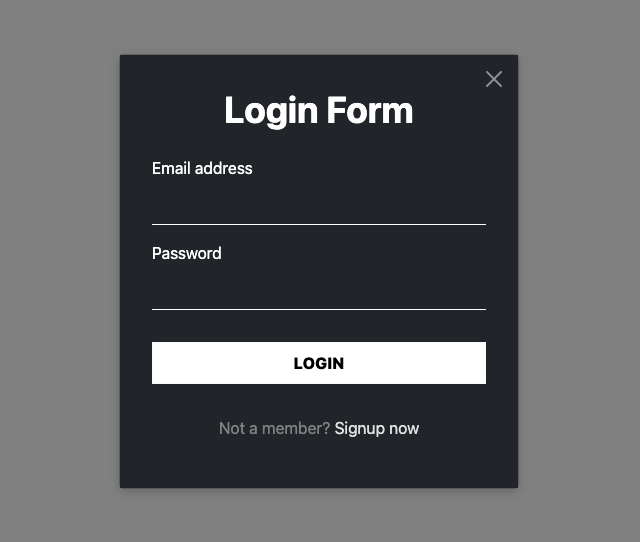
1. The form I am going to place in the Bootstrap modal is the Bootstrap Login Form, which I made some time ago.
<div class="myform bg-dark">
<h1 class="text-center">Login Form</h1>
<form>
<div class="mb-3 mt-4">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
</div>
<div class="mb-3">
<label for="exampleInputPassword1" class="form-label">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<button type="submit" class="btn btn-light mt-3">LOGIN</button>
<p>Not a member? <a href="#">Signup now</a></p>
</form>
</div>
Output:
Login Form
2. Let’s use this Bootstrap modal component.
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal">
Launch demo modal
</button>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
...
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
Output:
3. Add the form to the modal-body. Place the close button from the header inside the modal-body as well and remove the header and footer. (You can also try replacing the title in the header and signup option in the footer)
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal">
Launch demo modal
</button>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-body">
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
<div class="myform bg-dark">
<h1 class="text-center">Login Form</h1>
<form>
<div class="mb-3 mt-4">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
</div>
<div class="mb-3">
<label for="exampleInputPassword1" class="form-label">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<button type="submit" class="btn btn-light mt-3">LOGIN</button>
<p>Not a member? <a href="#">Signup now</a></p>
</form>
</div>
</div>
</div>
</div>
</div>
Output:
4. Let’s tweak it a bit to fit the modal. Set the max-width of the form to 100%.
.myform {
max-width: 100%;
}
Remove the default padding from modal-body.
.modal-body {
padding: 0;
}
Output:
5. Use Bootstrap class modal-dialog-centered to vertically center align the modal.
<div class="modal-dialog modal-dialog-centered">
Change the box-shadow as well.
.myform {
box-shadow: 0 4px 6px 0 rgba(22, 22, 26, 0.18);
}
Output:
6. To place the close button in the top right corner of the modal, set its position to absolute, and align it accordingly.
.btn-close {
position: absolute;
right: 0;
padding: 1em;
}
Since we have a dark background, use the white variant of the close button by adding the class btn-close-white.
<button type="button" class="btn-close btn-close-white" data-bs-dismiss="modal" aria-label="Close"></button>
Lastly, reduce the width of the modal and center align it.
.modal-content {
width: 80%;
margin: 0 auto;
}
Output:
Final Output Code for Responsive Bootstrap 5 Modal Form:
HTML
<button type="button" class="btn btn-dark" data-bs-toggle="modal" data-bs-target="#ModalForm">
Launch Modal Form
</button>
<div class="modal fade" id="ModalForm" tabindex="-1" aria-labelledby="ModalFormLabel" aria-hidden="true">
<div class="modal-dialog modal-dialog-centered">
<div class="modal-content">
<div class="modal-body">
<button type="button" class="btn-close btn-close-white" data-bs-dismiss="modal" aria-label="Close"></button>
<div class="myform bg-dark">
<h1 class="text-center">Login Form</h1>
<form>
<div class="mb-3 mt-4">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
</div>
<div class="mb-3">
<label for="exampleInputPassword1" class="form-label">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<button type="submit" class="btn btn-light mt-3">LOGIN</button>
<p>Not a member? <a href="#">Signup now</a></p>
</form>
</div>
</div>
</div>
</div>
</div>
CSS
.modal-content {
width: 80%;
margin: 0 auto;
}
.modal-body {
padding: 0;
}
.btn-close {
position: absolute;
right: 0;
padding: 1em;
}
h1 {
font-size: 2.3em;
font-weight: bold;
}
.myform {
padding: 2em;
max-width: 100%;
color: #fff;
box-shadow: 0 4px 6px 0 rgba(22, 22, 26, 0.18);
}
@media (max-width: 576px) {
.myform {
max-width: 90%;
margin: 0 auto;
}
}
.form-control:focus {
box-shadow: inset 0 -1px 0 #7e7e7e;
}
.form-control {
background-color: inherit;
color: #fff;
padding-left: 0;
border: 0;
border-radius: 0;
border-bottom: 1px solid #fff;
}
.myform .btn {
width: 100%;
font-weight: 800;
background-color: #fff;
border-radius: 0;
padding: 0.5em 0;
}
.myform .btn:hover {
background-color: inherit;
color: #fff;
border-color: #fff;
}
p {
text-align: center;
padding-top: 2em;
color: grey;
}
p a {
color: #e1e1e1;
text-decoration: none;
}
p a:hover {
color: #fff;
}
If you have any doubts or stuck somewhere, you can reach out through Coding Yaar's Discord server.